Learning Journey#4. Understanding REST APIs: for Beginners
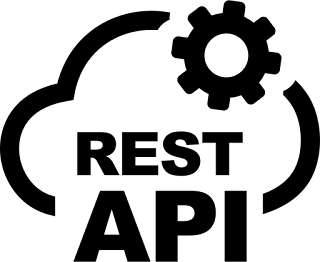
Welcome to the "Learning Journey" series. This space is my personal archive where I share insights and discoveries from my explorations into new tech territories, particularly back-end development and cloud services. As I continue to broaden my tech understanding, I hope this series inspires and contributes to your own learning journey. Introduction https://uxwing.com/rest-api-icon An API, or application programming interface, is a way for two pieces of software to communicate with each other. REST, or Representational State Transfer, is a set of architectural principles for designing APIs. REST APIs are the most common type of API and are employed in a wide array of applications, such as web browsers, mobile apps, and server-to-server communication. What is a REST API? A REST API is an API that adheres to the REST architectural principles. These principles dictate how